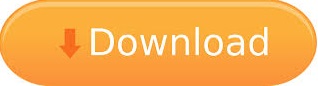
In this case, we want to allow only one thread (kid) to access the shared resource (iPad), so let’s set it to 1. This is the counter value that represents the number of threads we want to allow access to a shared resource at a given moment. In order to be consistent, this example will be based on the kids-iPad story.įirst, let’s create a semaphore instance: let semaphore = DispatchSemaphore(value: 1)ĭispatchSemaphore init function has one parameter called “value”. If the previous value is equal to or bigger than zero, it means the thread queue is empty, aka, no one is waiting.If the previous value was less than zero, this function wakes the oldest thread currently waiting in the thread queue.
#Geting semaphor value code
If the resulting value is equal to or bigger than zero, the code will get executed without waiting.If the resulting value is less than zero, the thread is frozen.We are basically signaling the semaphore that we are done interacting with the shared resource. Call signal() each time after using the shared resource.We are basically asking the semaphore if the shared resource is available or not.
Call wait() each time before using the shared resource. So, when should we call wait() and signal() functions? The counter value changes when we call signal() or wait() function. The counter value is used by the semaphore to decide if a thread should get access to a shared resource or not. The threads queue is used by the semaphore to keep track of waiting threads in FIFO order ( The first thread entered into the queue will be the first to get access to the shared resource once it is available). Thread-safe: code that can be safely called from multiple threads without causing any issues.Ī semaphore consists of a threads queue and a counter value (type Int). Such behavior could lead to race conditions, crashes, and obviously, our code won’t be thread-safe. If we compare this to programming, multiple threads try to access the same resource at the same time and nothing is preventing it. What if the father just gave the iPad to the kids? A fight would build up to the point of a probably broken iPad 😖. Tip: a shared resource can represent a variable, or a job such as downloading an image from a URL, reading from a database, etc. In addition, note the order of use, the first who asked is the first who get (FIFO). If we compare this to programming, only one thread has access to a shared resource at a time. Note how the father makes sure that only one kid uses the iPad at a time. In the scenario above, the father is the semaphore, the iPad is the shared resource, and the kids are the threads. Father: Kid 3, the iPad is available, let me know once you are done. Father: Kid 1, the iPad is available, let me know once you are done. Kid 2: I want to play with the iPad!!! Kid 1: NO!, I want to play first… Kid 3: Ipad! Ipad! Ipad! *sound of claps* Father: Ok, Kid 2, since you asked first and no one is currently using the iPad, take it, but let me know once you are done. For an easy start, let’s consider the following real-life scenario:Ī father sits with his three kids at home, then he pulls out an iPad… Semaphores give us the ability to control access to a shared resource by multiple threads.